Commits on Source (2)
Showing
- pulsjam2025/NPC/orca/orca.gd 115 additions, 50 deletionspulsjam2025/NPC/orca/orca.gd
- pulsjam2025/NPC/orca/orca.png 0 additions, 0 deletionspulsjam2025/NPC/orca/orca.png
- pulsjam2025/NPC/orca/orca.tscn 71 additions, 5 deletionspulsjam2025/NPC/orca/orca.tscn
- pulsjam2025/NPC/orca/orca.tscn6016116737.tmp 83 additions, 0 deletionspulsjam2025/NPC/orca/orca.tscn6016116737.tmp
- pulsjam2025/NPC/penguin/penguin.tscn 1 addition, 1 deletionpulsjam2025/NPC/penguin/penguin.tscn
- pulsjam2025/Player/PlayerScene.tscn 1 addition, 1 deletionpulsjam2025/Player/PlayerScene.tscn
- pulsjam2025/Player/TestScene.tscn 1 addition, 1 deletionpulsjam2025/Player/TestScene.tscn
- pulsjam2025/ice_floe/ice_floe.gd 0 additions, 1 deletionpulsjam2025/ice_floe/ice_floe.gd
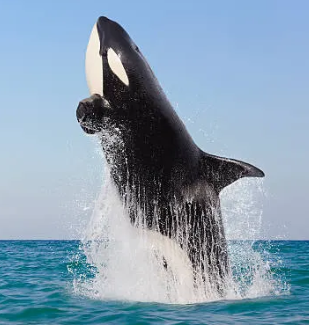
| W: | H:
| W: | H:
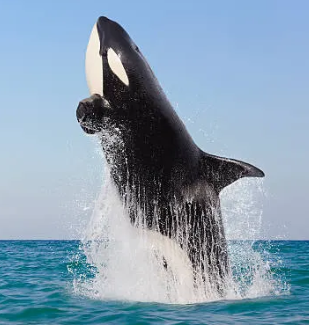
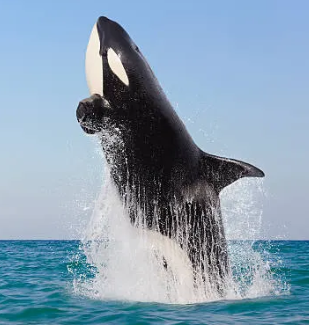
pulsjam2025/NPC/orca/orca.tscn6016116737.tmp
0 → 100644